jQuery is the easiest solution for DOM interactions. In this post, I am going to explain jQuery Basics. Next to that, I will show a live demo on code pen to demonstrate form interactions using jQuery Basics.
Before we start, lets understand what is the use of jQuery? In simple words, jQuery is an API to select DOM elements from HTML document. You can access/modify attributes on selected DOM elements and bind events to perform operation. Moreover, jQuery provides ajax API for server interactions without reloading page. jQuery provides methods for animation of DOM elements.
In this post, I am going to cover only how to manipulate DOM elements to access/modify its attributes and bind events.
jQuery Basics
jQuery has very simple fundamental.
- Basic 1: Select a DOM element
- Basic 2: Access/Modify selected DOM element’s attributes or Bind events.
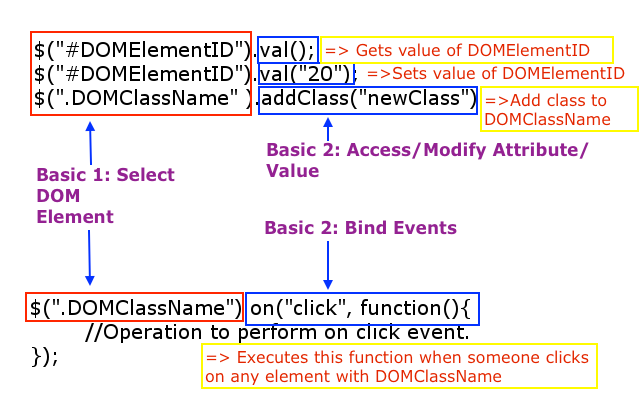
How to Select DOM Element?
jQuery provides multiple ways to select DOM elements.
Input Textbox
<input type="text" class="exampleClass"
id="exampleID" name="exampleName" value="20" />
-
“#” Selector to select DOM using ID of an element
$("#exampleID")
will select DOM element with ID = exampleID.$("#exampleID").val()
will return 20.$("#exampleID").val("Smooth Programming")
will set value to “Smooth Programming”. -
“.” Selector to select DOM using Class name of an element
$(".exampleClass")
will select DOM element with class = exampleClass.$(".exampleClass").val()
will return 20.$(".exampleClass").val("Smooth Programming")
will set value to “Smooth Programming”. -
“ElementName” Selector to select DOM using element name
$("input")
will select all input DOM elements.$("input").val()
will return 20.$("input").val("Smooth Programming")
will set value to “Smooth Programming”.
Input Radio Button
<input type="radio" id="genderMale" name="gender"
value="Male" checked> Male </input>
<input type="radio" id="genderFemale" name="gender"
value="Female"> Female </input>
-
“:checked” selector to get checked radio button
$("[name='gender']:checked").val()
will return “Male”. -
prop(“checked”, true/false) to Set radio button value
$("#genderFemale").prop("checked", true)
will mark Female value of radio button.$("#genderFemale").prop("checked", false)
will unmark Female value of radio button. -
is(“:checked”) to know radio button is checked or not?
$("#genderMale").is(":checked");
will return true because id#genderMale is checked.$("#genderFemale").is(":checked");
will return false because id#genderFemale is unchecked.
Input Checkbox
<input type="checkbox" id="hobbyCricket"
name="hobby" value="Cricket"> Cricket </input>
<input type="checkbox" id="hobbyBasketball"
name="hobby" value="Basketball" checked> Basketball </input>
<input type="checkbox" id="hobbyHockey"
name="hobby" value="Hockey" checked> Hockey </input>
<input type="checkbox" id="hobbyBadminton"
name="hobby" value="Badminton"> Badminton </input>
<input type="checkbox" id="hobbyFootball"
name="hobby" value="Football" checked> Football </input>
-
“:checked” to get FIRST CHECKED checkbox
$("[name='hobby']:checked").val()
will return “Basketball”. -
“.each()” function to iterate ALL CHECKED checkbox
$("[name='hobby']:checked").each(function(index){ console.log($(this).val()); }); // Output : Basketball, Hockey, Football
-
is(“:checked”) to know checkbox is checked or not?
$("#hobbyBasketball").is(":checked")
will return true because id#hobbyBasketball is checked.$("#hobbyCricket").is(":checked")
will return false because id#hobbyCricket is unchecked. -
attr(“checked”, true/false) to Set Checkbox value
$("#hobbyBasketball").attr("checked", true)
will mark ID#hobbyBasketball.$("#hobbyBasketball").attr("checked", false)
will unmark ID#hobbyBasketball.
Dropdown
<label> Select your highest level of Education. </label>
<select id="education" name="education" type="select">
<option value="0"></option>
<option value="1">Elementary</option>
<option value="2">High School</option>
<option value="3">Bachelor</option>
<option value="4" selected>Master</option>
<option value="5">PhD</option>
</select>
-
“option:selected” to get selected dropdown value
$("#education option:selected").val()
will return “4”.$("#education option:selected").text()
will return “Master”. -
.val() to select specific value in dropdown
$("#education").val("1")
will select “Elementary” option.
:checked
is used for radio buttons and checkboxes.
option:selected
is used for dropdown.jQuery Events
<form id="registration" method="post" action="#">
<label>Search Term</label>
<input id="name" type="text" value="" />
<input id="submitForm" type="submit" />
</form>
-
Bind submit event using
on
$("#registration").on("submit", function(event){ alert("Your name is :"+ $("#name").val()); event.preventDefault(); });
Above code will bind submit event to form with ID = registration.
-
Unbind submit event using
off
$("#registration").off("submit");
Above code will unbind submit event to form with ID = registration.
-
Other Frequently Used Events
.change() Binds change event handler to the change of an element.
.focus() Binds focus event handler to the focus of an element.
.submit() Binds focus event handler to the submit of form.
.click() Bind an event handler to the “click” JavaScript event, or trigger that event on an element.
.hover() Bind one or two handlers to the matched elements, to be executed when the mouse pointer enters and leaves the elements.
.trigger() Execute all handlers and behaviors attached to the matched elements for the given event type.
Live Demo
See the Pen jQuery Basic Form – Smooth Programming by Hiral Patel (@hiralbest) on CodePen.
Conclusion
Congratulations !!! You are good to go with DOM manipulations using jQuery. Please check jQuery Cheatsheet for quick reference to all most important jQuery operations.